Many-to-Many Relationships in MongoDB for Enhanced Database Management
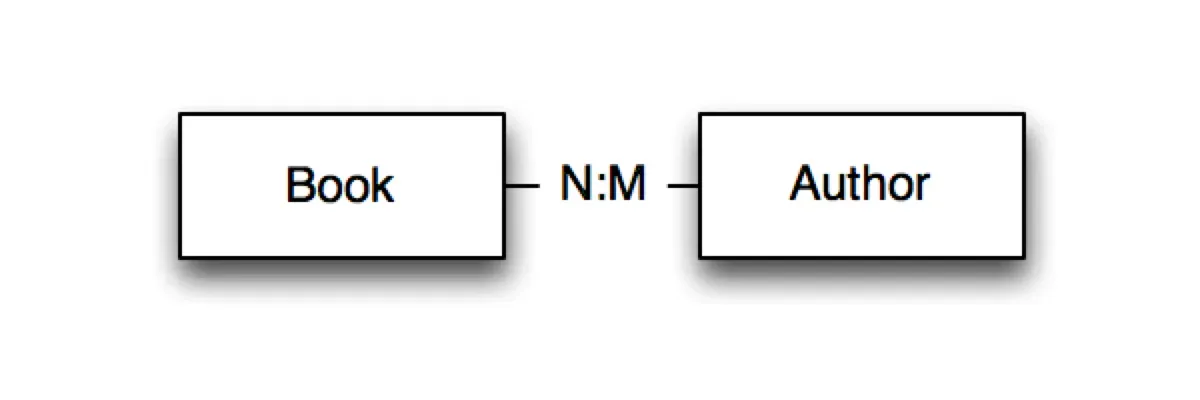
When working with MongoDB and NoSQL databases, one common challenge that often arises is how to model a many-to-many relationship efficiently. Unlike in traditional SQL databases where you can use linked tables to establish such relationships, MongoDB offers a different approach. In this article, we will explore how to tackle this issue and provide a solution that is both scalable and performant.
Understanding the Challenge
In the SQL world, many-to-many relationships are typically handled using linked tables, which serve as intermediaries between two or more related tables. However, in MongoDB, there are no direct equivalents to linked tables. Instead, MongoDB offers the option to use references (DBRefs) or embedding to establish relationships between collections.
Let’s consider an example to illustrate the challenge. Suppose we have two collections: Students
and Courses
. Students can enroll in multiple courses, and courses can have many students. In SQL, you might create a linked table to represent this relationship. In MongoDB, the initial approach might be to use references as follows:
const StudentSchema = new Schema({
name: String,
courses: [{ type: mongoose.Schema.Types.ObjectId, ref: 'Course' }]
});
const CourseSchema = new Schema({
name: String,
students: [{ type: mongoose.Schema.Types.ObjectId, ref: 'Student' }]
});
While this approach works, it can lead to scalability issues when dealing with large datasets. The arrays of students and courses within each document can grow significantly, impacting performance.
The Scalable Solution
To address the challenge of a many-to-many relationship in MongoDB and ensure scalability, we can adopt a de-normalized approach. This approach involves duplicating some data but optimizing for query performance.
Here’s how you can structure your collections to achieve this:
Student Collection:
{
_id: <student_id>,
name: <student_name>,
otherDetails: { ... },
courses: [
{ courseId: <course_id_1>, courseName: <course_name_1> },
{ courseId: <course_id_2>, courseName: <course_name_2> },
// ... (for all enrolled courses)
]
}
Course Collection:
{
_id: <course_id>,
name: <course_name>,
description: <course_description>,
otherDetails: { ... }
}
In this approach:
- Each student document includes an array of courses they are enrolled in, with each course represented as an embedded document.
- Each course document remains independent and contains its details.
Querying the Data
Now, let’s consider how you can perform common queries efficiently:
- Get all courses for a specific student:
db.students.find({ name: "John Doe" }, { courses: 1, name: 1 })
- Get all students enrolled in a specific course:
db.students.find({ "courses.courseName": "Database Design" })
This approach eliminates the need for a separate linked table and simplifies querying. Additionally, by de-normalizing the data, you reduce the need for complex queries and can take advantage of MongoDB’s native performance.
Conclusion
Handling many-to-many relationships in MongoDB requires a different mindset compared to traditional SQL databases. By adopting a de-normalized approach and embedding relevant data, you can achieve a scalable and efficient solution for managing relationships between collections. Remember that the specific design may vary based on your application's requirements, so always consider the nature of your data and the queries you need to perform when modeling your MongoDB schema.